I’m guessing that if you are reading this post you likely read the one before it where I talked about the virtues of implementing a MetaNav. In this post I’m going to talk about what you’re going to have to build in order to implement a MetaNav in your SharePoint 2010 (SP2010) Publishing site (aka: WCM site). At the core there are three major things you have to address:
- Navigation
- Rollup/Landing Pages & Controls
- Detail Pages
Building the Navigation Components
It wouldn’t be a MetaNav if you didn’t have navigation now, would it? Of course not. SP2010 implements navigation by leveraging the ASP.NET Navigation Provider framework. In this there are three main components:
- Navigation Provider - This piece is responsible for talking to some entity and creating the hierarchical collection of navigation nodes. Some out of the box providers include those that can look at an XML file or a SharePoint site. In our case we need to create one that looks at the SP2010 Managed Metadata Service (MMS) to extract a taxonomy.
- Data Source - The data source control is responsible for obtaining the navigation hierarchical structure from the navigation provider & figuring out what nodes should be included in the rendering… it essentially is filtering the nav. From here you do things like tell it to start at the top-most node or the current node, if the top-most node should be shown and things of that nature.
- Navigation Web Control - This control takes what the data source has filtered down and renders it out to HTML. From here you do things like control the CSS, indention and things of that nature.
As far as a MetaNav is concerned, there’s only one thing you need to build: a new navigation provider. As I said above, the job of this provider is to look at the taxonomy and build a collection of navigation nodes representing it. This is actually fairly easy to do as you’re just building an ASP.NET navigation provider.
Create a new class that inherits the PortalSiteMapProvider from the Publishing namespace and override just one method: GetChildNodes(). Then fill this method with the following code. This gets called for every node you create and it has the sole responsibility of returning the immediate child nodes under it. As you can see from the following class, I check to see if we’re at the top node and if we are, we start at the top of the taxonomy. Otherwise it’s a GUID, the ID of the term in the nav I created, I find the term in the term set & get all it’s children.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using Microsoft.SharePoint;
using Microsoft.SharePoint.Publishing.Navigation;
using Microsoft.SharePoint.Taxonomy;
using Microsoft.SharePoint.Publishing;
namespace CriticalPathTraining.SharePoint.Samples {
public class MetadataBasedNavProvider : PortalSiteMapProvider {
public override SiteMapNodeCollection GetChildNodes(SiteMapNode node) {
SiteMapNodeCollection navNodes = new SiteMapNodeCollection();
// cast it to a portal node from a regular .NET node
PortalSiteMapNode portalNode = node as PortalSiteMapNode;
// make sure it's a portal node
if (portalNode == null)
return navNodes;
// get refrerence to the taxonomy term store
TaxonomySession taxonomySession = new TaxonomySession(SPContext.Current.Site);
TermStore termStore = taxonomySession.TermStores\[0\];
Group termGroup = termStore.Groups\["Transportation"\];
TermSet termSet = termGroup.TermSets\["Automobile"\];
// site root
if (node.Key.ToLower() == SPContext.Current.Web.ServerRelativeUrl.ToLower()) {
foreach (var term in termSet.Terms) {
navNodes.Add(ProcessTerm(portalNode, term));
}
} else {
var subTerm = termSet.GetTerm(new Guid(node.Key));
foreach (var term in subTerm.Terms) {
navNodes.Add(ProcessTerm(portalNode, term));
}
}
return navNodes;
}
private SiteMapNode ProcessTerm(PortalSiteMapNode portalNode, Term termNode) {
var navNode = new PortalSiteMapNode(portalNode.WebNode,
termNode.Id.ToString(),
NodeTypes.Heading,
String.Format("{0}/Pages/rollup.aspx?tid={1}", SPContext.Current.Web.Url, termNode.Id),
termNode.Name,
string.Empty);
return navNode;
}
}
}
After building the project and deploying the DLL to the GAC, you next need to register the provider. Add the following to the element collection in the site’s web.config. This makes the site aware of the provider.
<add name="MetadataNavProvider"
type="CriticalPathTraining.SharePoint.Samples.MetadataBasedNavProvider,
CriticalPathTraining.SharePoint.Samples.MetadataBasedNavProvider,
Version=1.0.0.0, Culture=neutral, PublicKeyToken=31ad9365ecb382fd"
NavigationType="Global"
EncodeOutput="true" />
Lastly, you change the data source in the master page or where ever you are using it and poof, you got it working! From the pictures below, you can see the taxonomy…

Taxonomy in the term store
..and the nav:
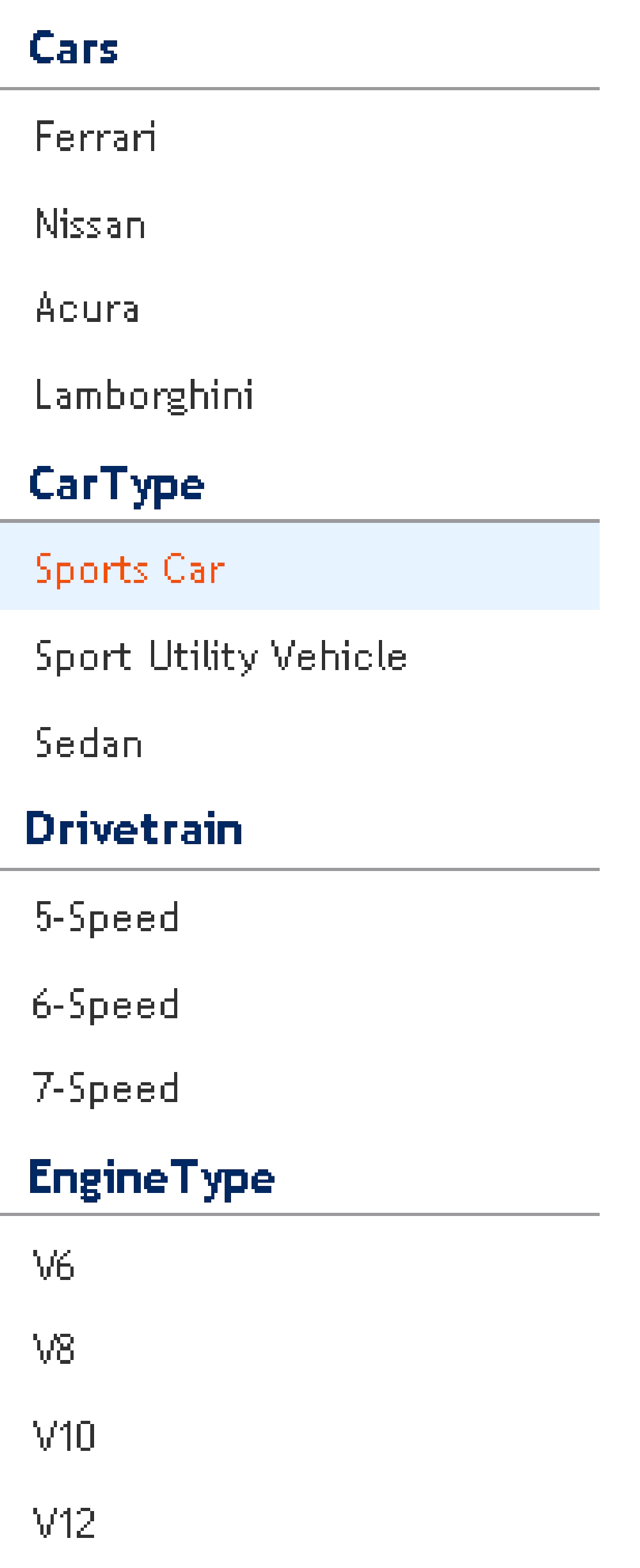
Taxonomy navigation
Building the Rollup/Landing Pages & Controls
Sometimes your navigation has the actual detail page links in them, but all the time your navigation points to rollup or landing pages. The goal of these rollup/landing pages is to present links to the other pages in your site. These pages (which could contain reusable controls) work best when you leverage search to find the pages that match the criteria on the landing page.
Below I’ve used a Web Part to show how to build the rollup. It is checking the taxid
QueryString parameter to find out what term was passed along. Here’s what it looks like:
using System;
using System.ComponentModel;
using System.Data;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using Microsoft.Office.Server.Search.Query;
using Microsoft.Office.Server.Search.Administration;
using Microsoft.SharePoint;
using Microsoft.SharePoint.Taxonomy;
namespace CriticalPathTraining.SharePoint.Samples.SearchTermRollupWebPart.SearchTermRollupWebPart {
[ToolboxItemAttribute(false)]
public class SearchTermRollupWebPart : WebPart {
protected override void CreateChildControls() {
// get term id from querystring
string taxid = this.Page.Request.QueryString\["tid"\].ToString();
// get the term name from MMS
// get refrerence to the taxonomy term store
TaxonomySession taxonomySession = new TaxonomySession(SPContext.Current.Site);
// get reference to first term store (can also get by name)
TermStore termStore = taxonomySession.TermStores\[0\];
Group termGroup = termStore.Groups\["Transportation"\];
TermSet termSet = termGroup.TermSets\["Automobile"\];
var matchingTerm = termSet.GetTerm(new Guid(taxid));
if (matchingTerm != null)
this.Controls.Add(new LiteralControl("" \+ matchingTerm.Name + "--------------------------"));
// execute query to get all pages matching the term
SearchServiceApplicationProxy proxy =
(SearchServiceApplicationProxy)SearchServiceApplicationProxy.GetProxy(
SPServiceContext.GetContext(SPContext.Current.Site)
);
KeywordQuery keywordQuery = new KeywordQuery(proxy);
keywordQuery.ResultsProvider = SearchProvider.Default;
keywordQuery.ResultTypes = ResultType.RelevantResults;
keywordQuery.EnableStemming = false;
keywordQuery.TrimDuplicates = true;
// create query
string pivotField = this.Page.Request.QueryString\["pt"\] != null ?
this.Page.Request.QueryString\["pt"\].ToString().ToLower() :
matchingTerm.Name.ToLower();
string query = "";
switch (pivotField) {
case "car type":
query = string.Format("{0}:{1}", ManagedProperties.CarType, taxid);
break;
case "drivetrain":
query = string.Format("{0}:{1}", ManagedProperties.Drivetrain, taxid);
break;
case "engine type":
query = string.Format("{0}:{1}", ManagedProperties.EngineType, taxid);
break;
case "make":
query = string.Format("{0}:{1}", ManagedProperties.Manufacturer, taxid);
break;
}
keywordQuery.QueryText = query;
ResultTableCollection searchResults = keywordQuery.Execute();
ResultTable relevantResults = searchResults\[ResultType.RelevantResults\];
DataTable relevantResultsTable = new DataTable();
relevantResultsTable.Load(relevantResults, LoadOption.OverwriteChanges);
// write results
foreach (DataRow searchResult in relevantResultsTable.Rows) {
this.Controls.Add(new LiteralControl("» "));
this.Controls.Add(new HyperLink() {
NavigateUrl = searchResult\[5\].ToString(),
Text = searchResult\[2\].ToString()
} );
this.Controls.Add(new LiteralControl(""));
}
}
}
}
Building Detail Pages
Generally there isn’t much of a problem in having to set this up. The pages are created like normal and the links to them will be visible in the search results. Thus when you create the rollup pages & controls, you’ll just link to these detail pages. However if you want to show content not just from your site collection, but also from other site collections If you want to show pages that don’t live in the site collection, such as those that live in another site collections or outside SharePoint. I doubt you’ll want to redirect your users from your site to wherever this content lives… you’ll likely want to surface the content in your site to maintain your user experience. One way to address this is to create your own version on the profile page capability that Business Connectivity Services (BCS) offers.